[ad_1]
Introduction
Algorithmic buying and selling is a broadly adopted buying and selling technique that has revolutionized the way in which folks commerce shares. An increasing number of individuals are earning profits on the aspect by investing in shares and automating their buying and selling methods. This tutorial will train you find out how to construct inventory buying and selling algorithms utilizing primitive technical indicators like MACD, SMA, EMA, and so on., and choose the very best methods primarily based on their precise efficiency/returns, fully utilizing Python.
Studying Aims
Get to know what algorithmic buying and selling is.
Construct easy inventory buying and selling algorithms in Python, utilizing technical indicators to generate purchase and promote alerts.
Study to implement buying and selling methods and automate them in Python.
Study to match and choose the very best buying and selling technique primarily based on their common returns.
This text was revealed as part of the Knowledge Science Blogathon.
Disclaimer – That is not monetary recommendation, and all work executed on this undertaking is for academic functions solely.
What’s Algorithmic Buying and selling?
Algorithmic buying and selling is a technique of buying and selling monetary belongings utilizing automated pc packages to execute orders primarily based on predefined guidelines and techniques. It includes the usage of a number of buying and selling methods, together with statistical arbitrage, pattern following, and imply reversion to call a number of.
There are lots of various kinds of algorithmic buying and selling. One among them is high-frequency buying and selling, which includes executing trades at excessive speeds with nearly no latency to benefit from small value actions. One other is news-based buying and selling, which includes making trades primarily based on information and different market occasions.
On this article, we can be utilizing Python to do inventory buying and selling primarily based on technical indicators and candlestick sample detection.
Use Python Algorithms for Inventory Buying and selling Evaluation?
We are able to analyze the inventory market, determine tendencies, develop buying and selling methods, and arrange alerts to automate inventory buying and selling – all utilizing Python! The method of algorithmic buying and selling utilizing Python includes a number of steps akin to choosing the database, putting in sure libraries, and historic knowledge extraction. Allow us to now delve into every of those steps and study to construct easy inventory buying and selling algorithms.
Deciding on the Dataset
There are literally thousands of publicly traded shares and we will take into account any set of shares for constructing the algorithm. Nonetheless, it’s at all times a very good possibility to contemplate related sorts of shares as their fundamentals and technicals can be comparable.
On this article, we can be contemplating the Nifty 50 shares. Nifty 50 index consists of fifty of the highest firms in India chosen primarily based on numerous components akin to market capitalization, liquidity, sector illustration, and monetary efficiency. The index can also be broadly used as a benchmark to measure the efficiency of the Indian inventory market and consequently, there’s a lesser quantity of danger concerned whereas investing in these firms when in comparison with investing in small-cap or mid-cap firms. I’ll take into account WIPRO for performing the evaluation on this article. The evaluation strategy mentioned on this article might be carried out on any set of comparable shares by calling the features for every inventory in a for loop.
Putting in the Required Libraries
We’ll use the default libraries like pandas, numpy, matplotlib together with yfinance and pandas_ta. The yfinance library can be used to extract the historic inventory costs. The pandas_ta library can be used for implementing the SMA and the MACD indicator and constructing the buying and selling algorithm. These modules might be straight put in utilizing pip like another Python library. Let’s import the modules after putting in them.
!pip set up yfinance
!pip set up pandas-ta
import yfinance as yf
import pandas as pd
import pandas_ta as ta
import numpy as np
from datetime import datetime as dt
import matplotlib.pyplot as plt
from datetime import timedelta as delta
import numpy as np
import os
import seaborn as sb
Now that we have now put in and imported all of the required libraries, let’s get our arms soiled and begin constructing the methods.
We’ll use the “obtain()” perform from the yfinance module to extract the historic value of a inventory, which accepts the inventory’s picker, begin date, and finish date. For the sake of simplicity, we are going to take “2000-01-01” as the beginning date and the present date as the tip date. We’ll write a easy perform that extracts the historic inventory costs and returns it as a knowledge body for processing additional whereas saving it as a CSV file on our disk.
def get_stock_info(inventory, save_to_disk=False):
start_date=”2000-01-01″
end_date = (dt.now() + delta(1)).strftime(‘%Y-%m-%d’)
df = yf.obtain(f”{inventory}.NS”, interval=’1d’, begin=start_date, finish=end_date, progress=False)
if(save_to_disk == True):
path=”./csv”
attempt: os.mkdir(path)
besides OSError as error: cross
df.to_csv(f'{path}/{inventory}.csv’)
return df
df = get_stock_info(‘WIPRO’, save_to_disk = True)
Constructing Inventory Buying and selling Algorithms Utilizing Technical Indicators
There are quite a few indicators out there for performing inventory buying and selling however we can be utilizing one of many two easiest but extraordinarily widespread indicators specifically, SMA and MACD. SMA stands for Easy Transferring Common whereas MACD stands for Transferring Common Convergence Divergence. In case you aren’t conversant in these phrases, you possibly can study extra about them on this article. In brief, we are going to attempt to discover the SMA crossover and MACD crossover as commerce alerts and attempt to discover the very best mixture of the lot for maximized returns.
For the SMA crossover, we are going to take the 10-day, 30-day, 50-day, and 200-day transferring averages under consideration. For the MACD crossover, we are going to take the 12-day, 26-day, and 9-day exponential transferring averages under consideration. Let’s calculate these values utilizing the pandas_ta library.
For calculating the SMA, we are going to use the “sma()” perform by passing the adjusted shut value of the inventory together with the variety of days. For calculating the MACD, we are going to use the “macd()” perform by passing the adjusted shut value of the inventory and setting the quick, sluggish, and sign parameters as 12, 26, and 9 respectively. The SMA and MACD values don’t make quite a lot of sense as such. So, let’s encode them to grasp if there are any crossovers.
Within the case of SMA, we are going to take 3 circumstances:
The ten-day SMA needs to be above the 30-day SMA.
The ten-day and 30-day SMA needs to be above the 50-day SMA.
The ten-day, 30-day, and 50-day needs to be above the 200-day SMA.
Within the case of MACD, we may have 2 circumstances:
The MACD needs to be above the MACD sign.
The MACD needs to be higher than 0.
The Python code given beneath creates a perform to implement the circumstances talked about above.
def add_signal_indicators(df):
df[‘SMA_10’] = ta.sma(df[‘Adj Close’],size=10)
df[‘SMA_30’] = ta.sma(df[‘Adj Close’],size=30)
df[‘SMA_50’] = ta.sma(df[‘Adj Close’],size=50)
df[‘SMA_200’] = ta.sma(df[‘Adj Close’],size=200)
macd = ta.macd(df[‘Adj Close’], quick=12, sluggish=26, sign=9)
df[‘MACD’] = macd[‘MACD_12_26_9’]
df[‘MACD_signal’] = macd[‘MACDs_12_26_9’]
df[‘MACD_hist’] = macd[‘MACDh_12_26_9′]
df[’10_cross_30’] = np.the place(df[‘SMA_10’] > df[‘SMA_30’], 1, 0)
df[‘MACD_Signal_MACD’] = np.the place(df[‘MACD_signal’] < df[‘MACD’], 1, 0)
df[‘MACD_lim’] = np.the place(df[‘MACD’]>0, 1, 0)
df[‘abv_50’] = np.the place((df[‘SMA_30’]>df[‘SMA_50’])
&(df[‘SMA_10’]>df[‘SMA_50’]), 1, 0)
df[‘abv_200’] = np.the place((df[‘SMA_30’]>df[‘SMA_200’])
&(df[‘SMA_10’]>df[‘SMA_200’])&(df[‘SMA_50’]>df[‘SMA_200’]), 1, 0)
return df
df = add_signal_indicators(df)
Now that we have now all of the alerts added to our knowledge, it’s time to calculate the returns. The returns can be crucial side for choosing the right buying and selling technique amongst the lot. We’ll calculate the 5-day and 10-day returns of the inventory. We may even label encode the returns as 0 and 1 with 0 indicating detrimental returns and 1 indicating constructive returns. Let’s go forward and create the perform implementing the identical.
def calculate_returns(df):
df[‘5D_returns’] = (df[‘Adj Close’].shift(-5)-df[‘Adj Close’])/df[‘Close’]*100
df[’10D_returns’] = (df[‘Adj Close’].shift(-10)-df[‘Adj Close’])/df[‘Close’]*100
df[‘5D_positive’] = np.the place(df[‘5D_returns’]>0, 1, 0)
df[’10D_positive’] = np.the place(df[’10D_returns’]>0, 1, 0)
return df.dropna()
df = calculate_returns(df)
Understanding the Efficiency of the Alerts
We are able to take all of the circumstances talked about above and carry out a easy combination to calculate the typical and the median returns we will anticipate whereas buying and selling primarily based on these alerts. We are able to additionally extract the minimal and the utmost returns every sign has generated up to now. This won’t solely give us a tough understanding of how good the alerts are but additionally an concept of how a lot returns might be anticipated whereas buying and selling utilizing these alerts. Let’s write a easy code to do the identical utilizing Python.
def get_eda_and_deepdive(df):
eda = df.dropna().groupby([’10_cross_30′, ‘MACD_Signal_MACD’,
‘MACD_lim’, ‘abv_50’, ‘abv_200’])[[‘5D_returns’, ’10D_returns’]]
.agg([‘count’, ‘mean’,’median’, ‘min’, ‘max’])
deepdive = df.dropna().groupby([’10_cross_30′, ‘MACD_Signal_MACD’,
‘MACD_lim’, ‘abv_50’, ‘abv_200′,’5D_positive’, ’10D_positive’])[[‘5D_returns’, ’10D_returns’]]
.agg([‘count’, ‘mean’,’median’, ‘min’, ‘max’])
return eda, deepdive
eda, deepdive = get_eda_and_deepdive(df)
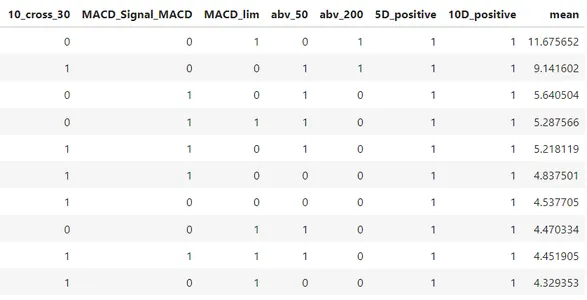
Let’s visualize the field whiskers plot for the highest 10 sign combos sorted primarily based on the 5-day and the 10-day returns.
x = df.copy()
def _fun(x):
code=””
for i in x.keys(): code += str(x[i])
return code
x[‘signal’] = x[[’10_cross_30′, ‘MACD_Signal_MACD’, ‘MACD_lim’, ‘abv_50’, ‘abv_200’,
‘5D_positive’, ’10D_positive’]].apply(_fun, axis=1)
x = x.dropna()
lim = x.groupby([’10_cross_30′, ‘MACD_Signal_MACD’, ‘MACD_lim’,
‘abv_50’, ‘abv_200’, ‘5D_positive’, ’10D_positive’])[‘5D_returns’].agg([‘mean’]).reset_index()
lim = lim.sort_values(by=’imply’, ascending=False).head(10)
x = x.merge(lim, on=[’10_cross_30′, ‘MACD_Signal_MACD’, ‘MACD_lim’,
‘abv_50’, ‘abv_200’, ‘5D_positive’, ’10D_positive’], how=’internal’)
ax = sb.boxplot(x=’sign’, y=’5D_returns’, knowledge=x)
ax.set_xticklabels(ax.get_xticklabels(), rotation=45)
plt.present()
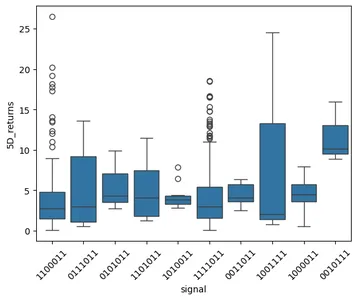
ax = sb.boxplot(x=’sign’, y=’10D_returns’, knowledge=x)
ax.set_xticklabels(ax.get_xticklabels(), rotation=45)
plt.present()
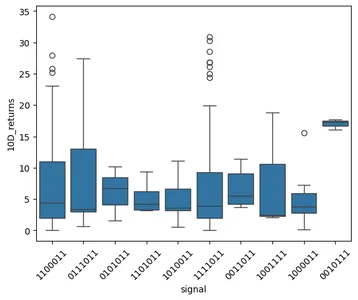
Taking solely the 5-day and 10-day returns for choosing the right alerts shouldn’t be the very best strategy as a result of we are going to by no means know what number of occasions the sign has given constructive returns towards detrimental returns. This strategy could possibly be taken under consideration whereas choosing the right methods, which may doubtlessly enhance the efficiency of the methods. I cannot take this strategy to maintain this text easy and beginner-friendly.
Conclusion
The idea of algorithmic buying and selling might be extraordinarily tempting for a lot of as it may be very profitable however on the similar time, it tends to be an especially complicated and tedious activity to construct inventory buying and selling algorithms from scratch. It is extremely essential to grasp the truth that all algorithms can fail, which may doubtlessly result in large monetary losses when deployed to a dwell buying and selling surroundings. The goal of this text was to discover how easy buying and selling algorithms might be constructed and validated utilizing Python. To proceed additional on this undertaking, chances are you’ll take into account different technical indicators and candlestick patterns, and use them interchangeably to construct extra complicated algorithms and techniques.
Key Takeaways
On this article, we realized to extract historic inventory costs utilizing yfinance.
We realized to calculate MACD and SMA values primarily based on the inventory value and construct Python algorithms to create alerts/methods utilizing these values.
We additionally realized to calculate and visualize the 5-day and 10-day returns of the methods on the inventory.
Word: This isn’t monetary recommendation, and all work executed on this undertaking is for academic functions solely. That’s it for this text. Hope you loved studying this text and realized one thing new. Thanks for studying and blissful studying!
Often Requested Questions
Ans. No, all inventory buying and selling methods are sure to fail and may result in capital loss. The methods used on this article are for academic functions solely. Don’t use them to make monetary investments.
Ans. Sure, these alerts might be thought of feature-engineered variables and can be utilized for performing machine studying or deep studying.
Ans. It is very important choose related shares as their fundamentals and different parameters like alpha/beta can be comparable. The alpha and beta values of a large-cap firm and a small-cap firm could be in several ranges. Therefore, it may not be proper to randomly combine them whereas performing this evaluation.
Ans. There are tons of of indicators out there and broadly used for buying and selling like RSI, MACD, and SMA. There are quite a few technical candlestick indicators out there as effectively like HARAMICROSS, MORNINGSTAR, and HAMMER that may assist generate the alerts.
Ans. This evaluation might be carried out for commodities with huge historic knowledge. However within the case of cryptocurrencies, it depends upon how a lot historic knowledge is really out there. The evaluation would possibly fail or give a improper conclusion if the alerts happen a really occasions in your complete historic knowledge.
The media proven on this article shouldn’t be owned by Analytics Vidhya and is used on the Writer’s discretion.
Associated
[ad_2]
Source link
Избранные трендовые новости часового мира – последние новинки лучших часовых марок.
Все коллекции часов от дешевых до экстра люксовых.
https://watchco.ru/
Все актуальные новости часового искусства – актуальные новинки именитых часовых брендов.
Абсолютно все коллекции хронографов от недорогих до экстра люксовых.
https://bitwatch.ru/